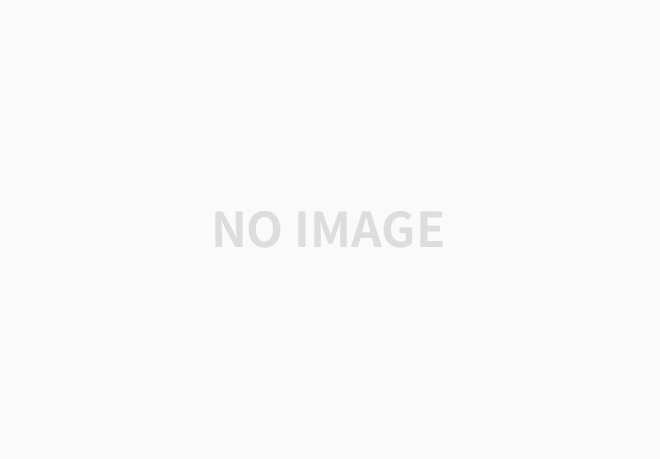
Label
에 대해 알아보도록 합시다.
Label
Label("some string", systemImage: "some SF Symbols")
Label { Text("some text") } icon : { Image(systemName: "some image") }
SwiftUI에서 가장 일반적이고 인식 가능한 User interface
의 구성 요소 중 하나는 아이콘과 레이블의 조합입니다.
이 관용구는 여러 종류의 앱에 사용되고 collection
, list
, menu
의 액션 아이템 등에 표시됩니다.
그러면 SF Symbols
를 사용하여 간단한 형식의 레이블을 만들어 보도록 하겠습니다.
List { //1. 기본적인 레이블 Label("나의 계정", systemImage: "person.crop.circle")
//2. 제목만 표시하는 레이블 스타일 Label("Wi-Fi", systemImage: "wifi") .labelStyle(TitleOnlyLabelStyle())
//3. 아이콘만 표시하는 레이블 스타일 Label("Wi-Fi", systemImage: "wifi") .labelStyle(IconOnlyLabelStyle())
//4. 레이블 크기 변경 Label("개인용 핫스팟", systemImage: "personalhotspot") //.font(.largeTitle) .font(.system(size: 30))
//5. 텍스트와 이미지 각각 커스튬 Label { Text("에어팟") .foregroundColor(Color.orange) } icon : { Image(systemName: "airpodspro") .foregroundColor(Color.blue) }
//6. 사용자 이미지 사용 Label { Text("서근 개발블로그") .font(.system(size: 16)) } icon : { Image("welcome") .resizable() .scaledToFit() .frame(width: 30) }
//7. 라벨 이미지 대신 도형넣기 Label { Text("서근 개발블로그") .foregroundColor(.blue) } icon : { Capsule().frame(width: 20, height: 30) .foregroundColor(.black) }
//8. 라벨 이미지 대신 도형넣기-2 Label { Text("서근") .foregroundColor(.primary) .font(.largeTitle) .padding() .background(Color.yellow.opacity(0.3)) .clipShape(Capsule()) .padding(-10) } icon: { RoundedRectangle(cornerRadius: 32) .fill(Color.blue) .frame(width: 64, height: 64) }
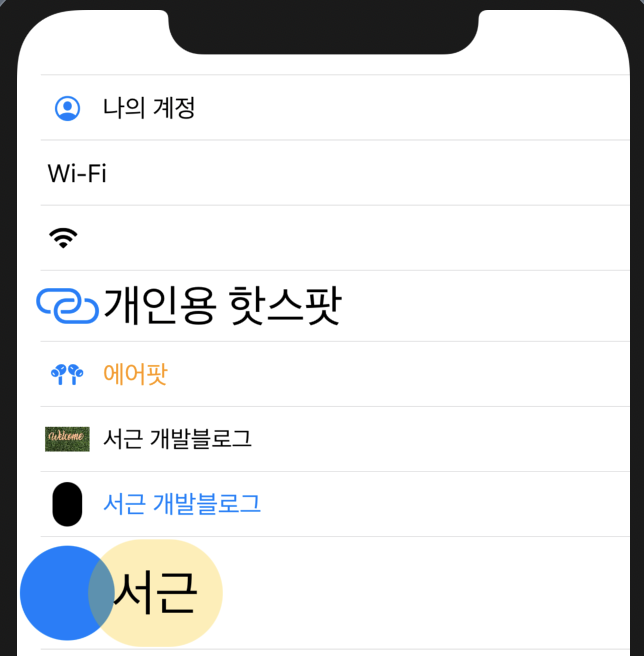
Label style
기본 Label Style 종류
TitleAndIconLabelStyle()
iOS 14.5에서만 사용할 수 있습니다.
사용자 지정
레이블을 완전하게 사용자 지정하거나 완전하게 새로운 레이블 Style
을 만드려면 LabelStyle
프로토콜을 구현해야 합니다.
struct MyCustomLabelStyle: LabelStyle { func makeBody(configuration: Configuration) -> some View { // Your custom code here } }
이러한 기본값에 사용자가 원하는 코드를 추가해줘야 합니다.
레이블 배경색 추가 (Label Style)
레이블 주변에 padding
을 추가하고 배경색을 원한는 색으로 만드는 프로토콜을 만들어 보겠습니다.
레이블 배경색 Code
//사용자 레이블 스타일 생성 struct YellowBackgroundLabelStyle: LabelStyle { func makeBody(configuration: Configuration) -> some View { Label(configuration) .padding() .background(Color.yellow) .cornerRadius(20) } }
이제 이 LabelStyle
을 적용 시켜주겠습니다.
//9. 사용자 레이블 스타일 적용 Label("사용자 레이블 스타일 지정 (1)", systemImage: "paperplane.circle.fill") .labelStyle(YellowBackgroundLabelStyle()) //9-1 Label { Text("사용자 레이블 스타일 지정 (2)") } icon : { Image(systemName: "paperplane.circle.fill") } //레이블스타일은 레이블 밖에서 작성해야한다. .labelStyle(YellowBackgroundLabelStyle())
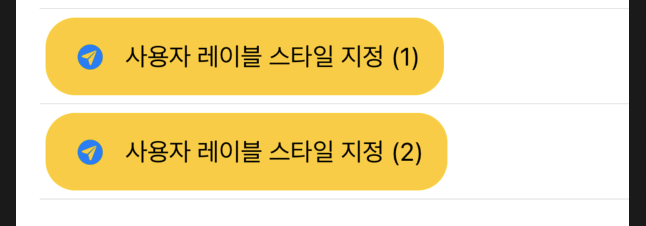
Vertical 레이블 추가 (Label Style)
레이블 요소를 다시 정렬하여 수직으로 쌓아 올리는 스타일을 만들어 보도록 하겠습니다.
VStack
을 사용해서 뷰를 구현 할 수 있습니다.
Vertical 레이블 Code
// 사용자 레이블 스타일 생성 - Vertical 레이블 스타일 생성 struct VerticalLabelStyle: LabelStyle { func makeBody(configuration: Configuration) -> some View { VStack { configuration.icon .padding(5) configuration.title } } }
이제 이 LabelStyle
을 적용 시켜주겠습니다.
//10. vertical 레이블 Label { Text("수직 레이블뷰") } icon : { Image(systemName: "moon.fill") } .labelStyle(VerticalLabelStyle())

아이콘에 컬러를 추가해주는 레이블 추가 (Label Style)
아이콘 안에 컬러를 추가해주는 레이블 스타일
을 만들어 주겠습니다.
Colorful Icon 레이블 Code
struct Settings: View { //반드시 CGFloat을 추가해줘야 합니다. @ScaledMetric var size: CGFloat = 1 var body: some View { }
// 사용자 레이블 스타일 생성 - Colorful아이콘 스타일 struct ColorfulIconLabelStyle: LabelStyle { var color: Color var size: CGFloat func makeBody(configuration: Configuration) -> some View { Label { configuration.title } icon: { configuration.icon .imageScale(.small) .foregroundColor(.white) .background(RoundedRectangle(cornerRadius: 7 * size).frame(width: 28 * size, height: 28 * size).foregroundColor(color)) } } }
이제 이 LabelStyle
을 적용 시켜주겠습니다.
Section { //11. 아이콘에 색상 추가 Label("Airplane Mode", systemImage: "airplane").labelStyle(ColorfulIconLabelStyle(color: .orange, size: size)) Label("Wi-Fi", systemImage: "wifi").labelStyle(ColorfulIconLabelStyle(color: .blue, size: size)) Label("Bluetooth", systemImage: "airpodspro").labelStyle(ColorfulIconLabelStyle(color: .blue, size: size)) Label("Cellular", systemImage: "antenna.radiowaves.left.and.right").labelStyle(ColorfulIconLabelStyle(color: .green, size: size)) Label("Personal Hotspot", systemImage: "personalhotspot").labelStyle(ColorfulIconLabelStyle(color: .green, size: size)) } Section { Label("Notifications", systemImage: "app.badge").labelStyle(ColorfulIconLabelStyle(color: .red, size: size)) Label("Sounds & Haptics", systemImage: "speaker.wave.3.fill").labelStyle(ColorfulIconLabelStyle(color: .red, size: size)) Label("Do not disturb", systemImage: "moon.fill").labelStyle(ColorfulIconLabelStyle(color: .purple, size: size)) Label("Display time", systemImage: "hourglass").labelStyle(ColorfulIconLabelStyle(color: .purple, size: size)) }
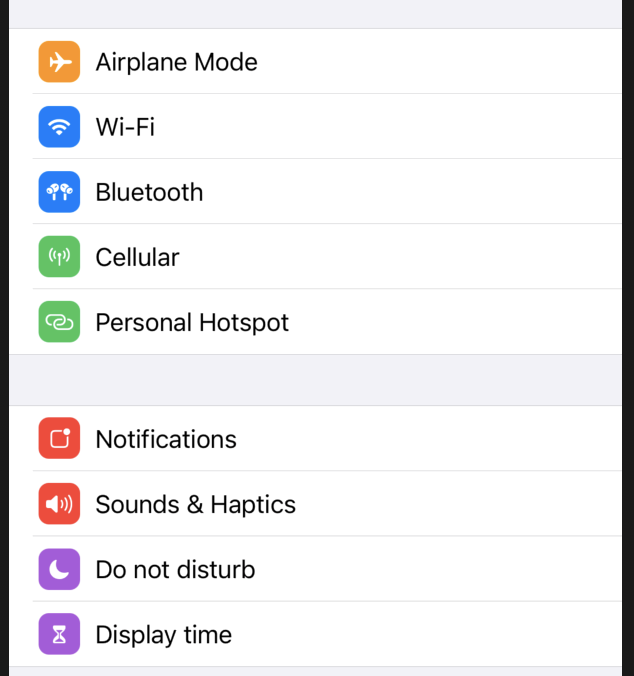
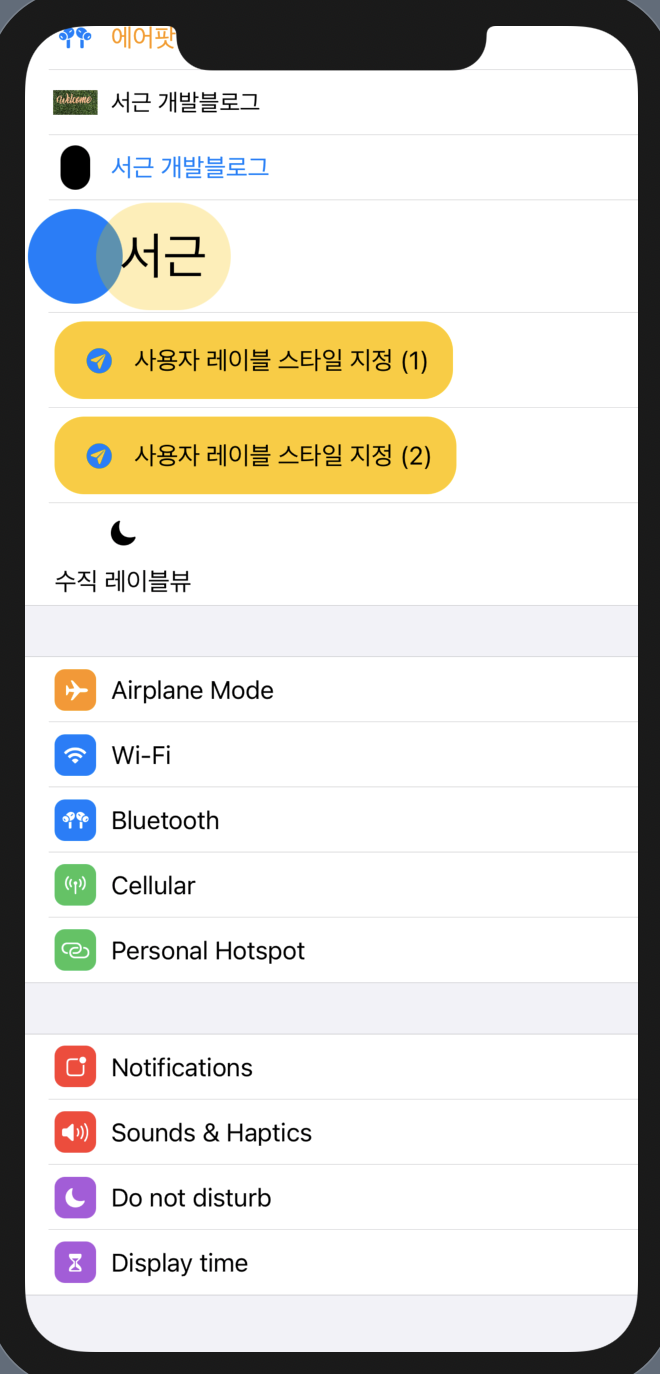
읽어주셔서 감사합니다🤟
본 게시글의 전체코드 GitHub 👇🏻
Seogun95/SwiftUI_Label_TUT
Label과 LabelStyle에 대해 알아봅시다. Contribute to Seogun95/SwiftUI_Label_TUT development by creating an account on GitHub....
github.com
참고하면 좋은 게시글👇🏻
SwiftUI : List Style
본 게시물은 Zedd님의 게시물을 인용하여 작성되었습니다. List Style에 대해 알아보도록 합시다. listStyle을 사용하기위해서는 List { ... } .listStyle(____()) 처럼 리스트 밖에 스타일을 추가해줘야 합니...
seons-dev.tistory.com
'SWIFTUI > View layout' 카테고리의 다른 글
SwiftUI : ColorPicker (0) | 2021.02.07 |
---|---|
SwiftUI : ProgressView (작업 진행률) 타이머 (0) | 2021.02.07 |
SwiftUI : Lazy V(H)Stack (0) | 2021.02.06 |
SwiftUI : SearchView (0) | 2021.02.03 |
SwiftUI : Slider (0) | 2021.02.01 |